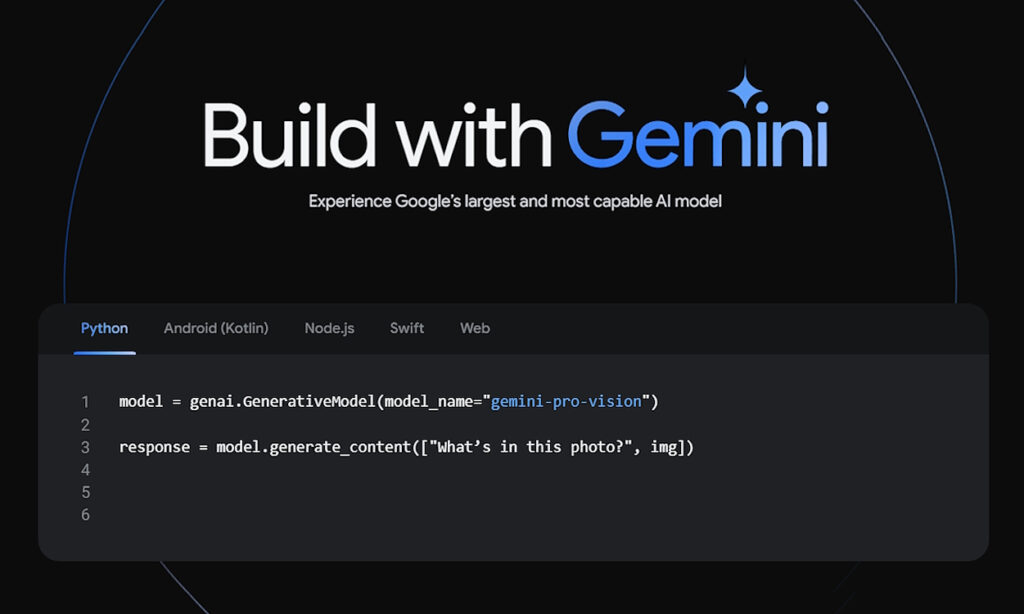
Following Gemini AI’s announcement, Google has now made its Gemini models accessible through API. Presently, the company provides API access to Gemini Pro, encompassing both text-only and text-and-vision models. This release marks a significant addition as, up to this point, Google had not incorporated visual capabilities into Bard, which operated solely on the text-only model. With this API key, users can promptly test Gemini’s multimodal functions on their local computer. In this guide, we’ll delve into accessing and utilizing the Gemini API.
Note: The Google Gemini API key is currently offered for free for both text and vision models. This complimentary access will remain in effect until the general availability slated for early next year. During this period, users can send up to 60 requests per minute without the need to configure Google Cloud billing or incurring any expenses.
Setting Up Python and Pip on Your Computer
- Access our guide and install Python along with Pip on your PC or Mac. Ensure you install Python version 3.9 or above.
- For Linux users, follow our tutorial to install Python and Pip on Ubuntu or other distributions.
- In the Terminal, execute the commands below to confirm the installation of Python and Pip on your computer. These commands should display the version numbers.
python -V
pip -V
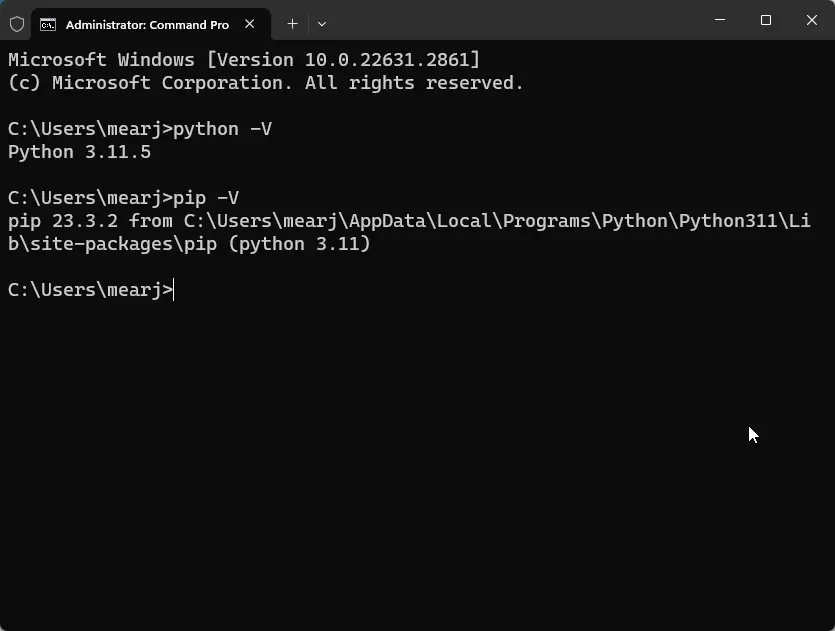
- Upon successful installation, use the command below to install Google’s Generative AI dependency.
pip install -q -U google-generativeai
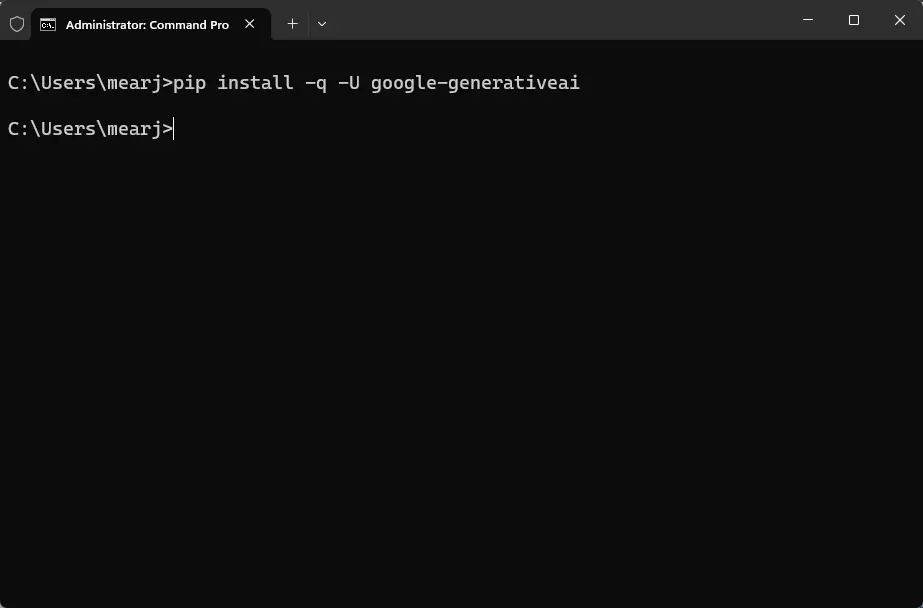
How to Acquire the Gemini Pro API Key
- Visit makersuite.google.com/app/apikey (visit) and log in using your Google account.
- Click the “Create API key in new project” option within the API keys section.
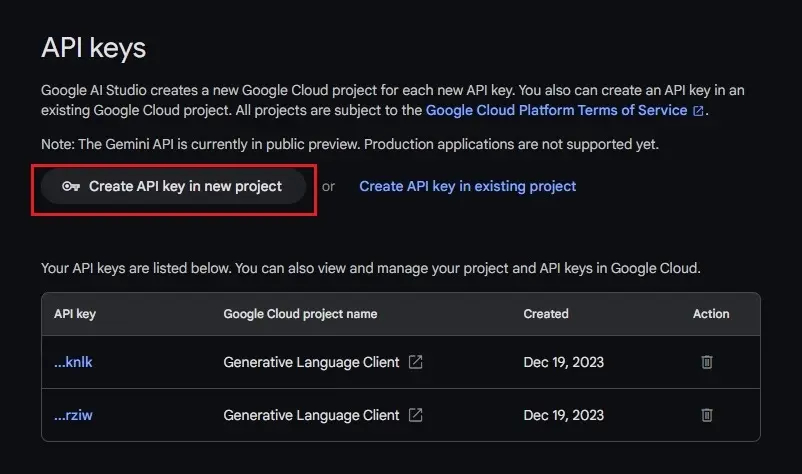
- Once generated, copy the API key and refrain from sharing it publicly to maintain its confidentiality.
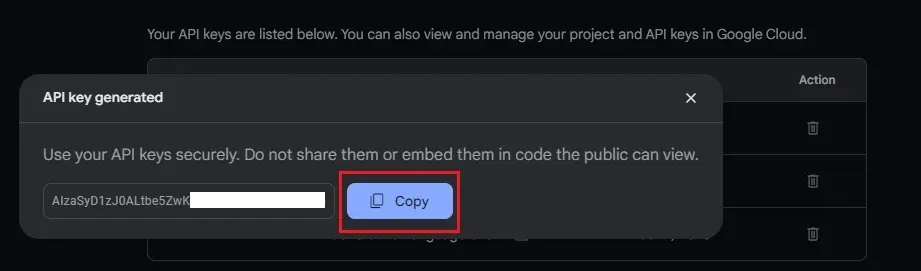
How to Utilize the Gemini Pro API Key (Text-only Model)
Just like OpenAI, Google has streamlined the process of using its Gemini API key for development and testing. I’ve simplified the code to make it accessible for anyone looking to test and use it. In this example, I’ll showcase how to access the Gemini Pro Text model via the API key.
- Choose a Code Editor: If you’re a beginner, consider installing Notepad++ (visit). Advanced users might prefer Visual Studio Code (visit).
- Copy and Paste the Code: Paste the provided code into your chosen code editor.
import google.generativeai as genai
genai.configure(api_key='PASTE YOUR API KEY HERE')
model = genai.GenerativeModel('gemini-pro')
response = model.generate_content("What is the meaning of life?")
print(response.text)
- Insert Your API Key: Replace ‘PASTE YOUR API KEY HERE’ with your actual Gemini API key. The code defines the ‘gemini-pro‘ model for text generation and includes a query prompt.
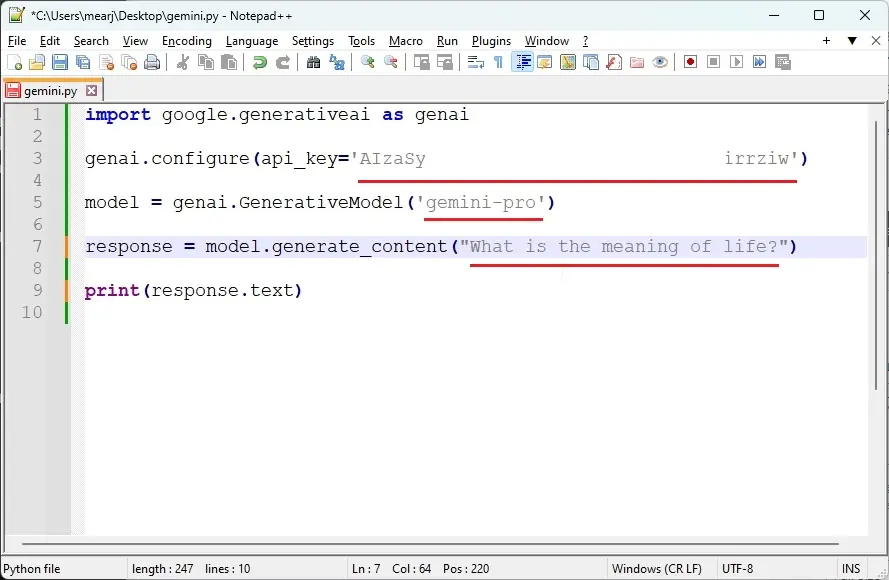
- Save the Code: Save the code with a name ending in
.py.
For instance, you can name itgemini.py
and save it to your Desktop.
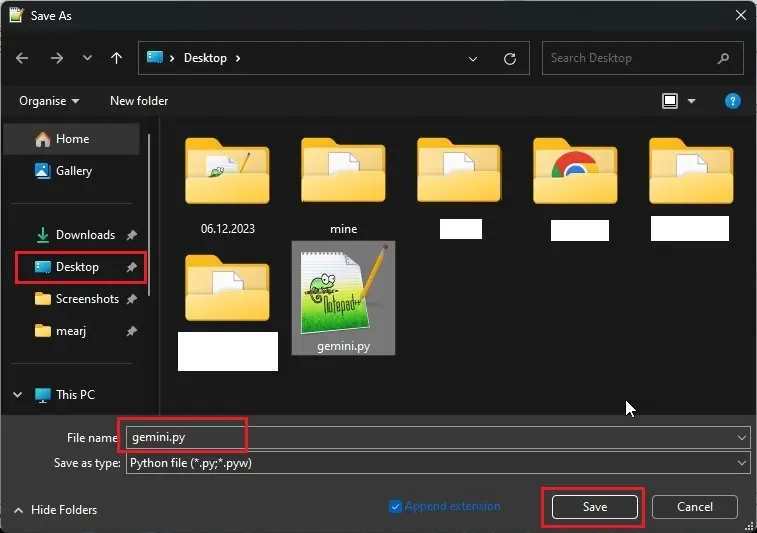
- Access the Terminal: Open the Terminal and navigate to the Desktop using the command:
cd Desktop
- Execute the Python Script: Run the Python script
gemini.py
using the following command:
python gemini.py
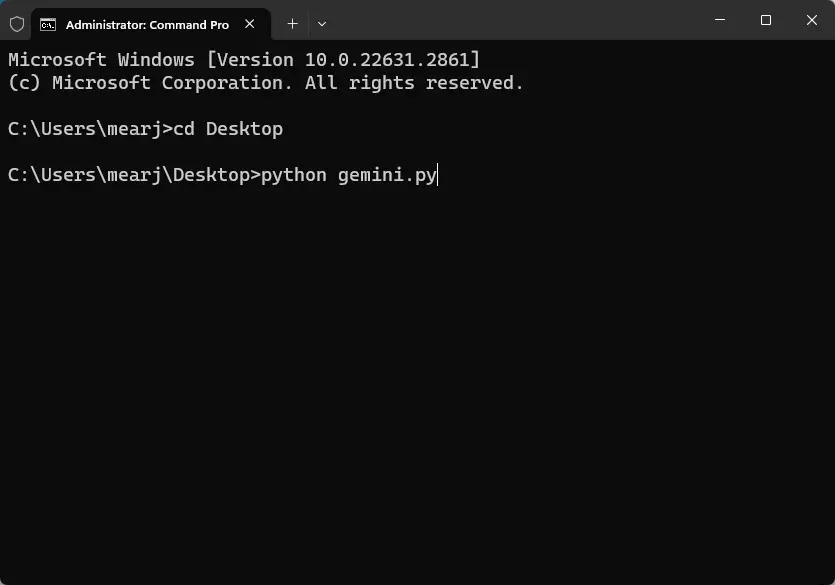
- Get the Response: The script will answer the question specified in the
gemini.py
file and display the response in the Terminal.
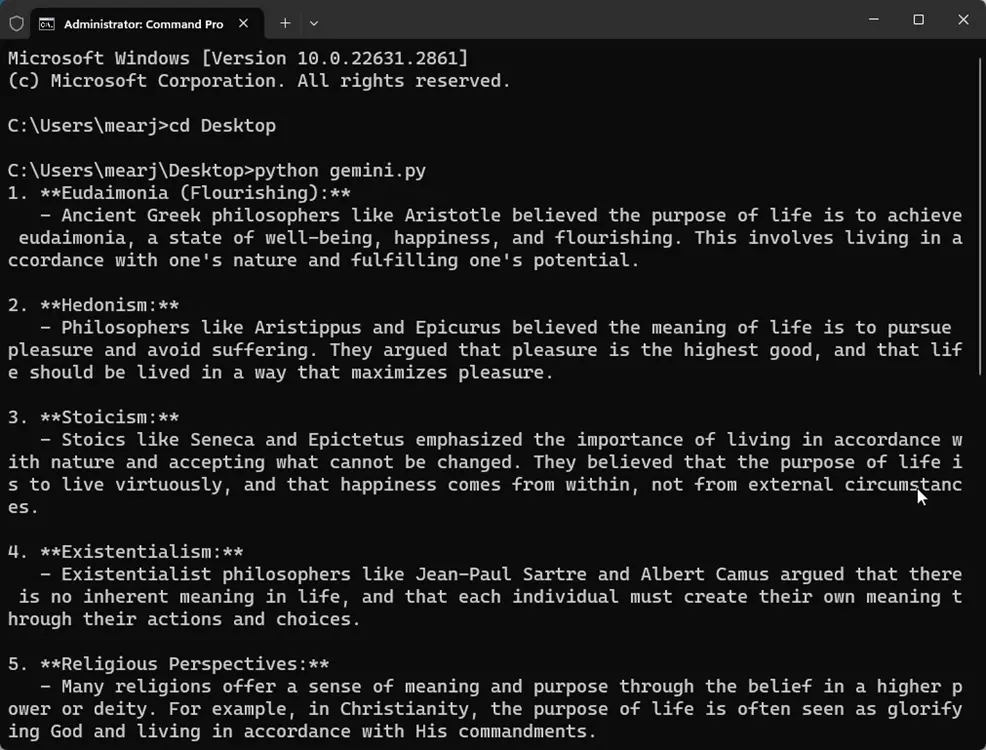
- Try Different Queries: Edit the question in the code editor, save it, and rerun the
gemini.py
file in the Terminal to receive a new response. This demonstrates how you can leverage the Google Gemini API key to access the text-only Gemini Pro model for various inquiries.
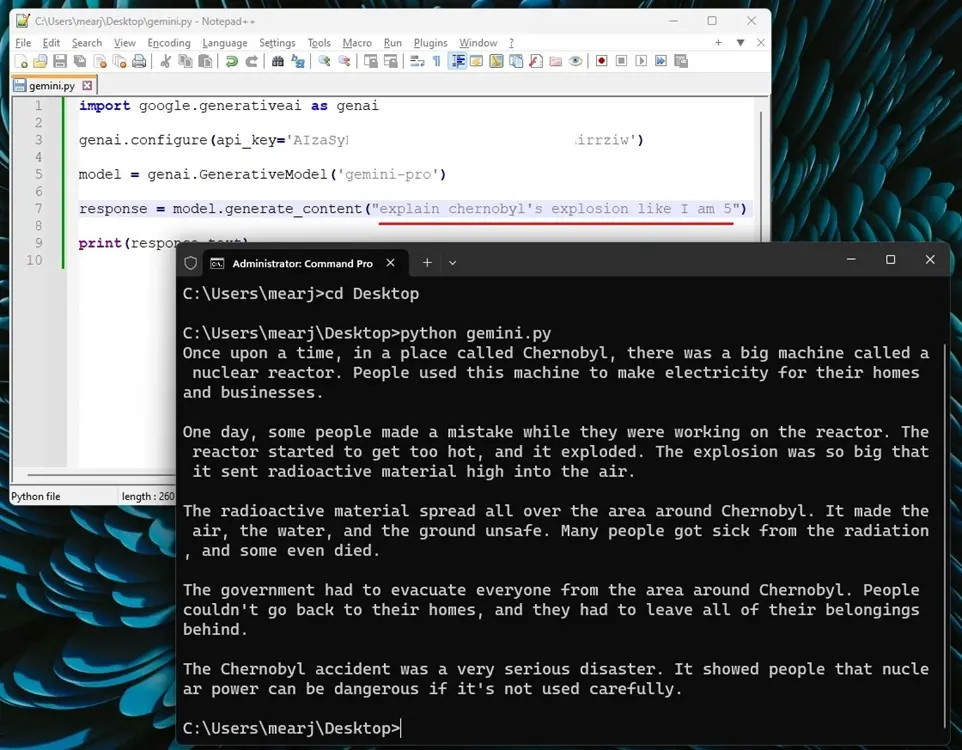
How to Utilize the Gemini Pro API Key (Text-and-Vision Model)
In this demonstration, I’ll illustrate how you can engage with the Gemini Pro multimodal model. While it’s not yet available on Google Bard, you can access it instantly through the API. Fortunately, the process remains straightforward and effortless.
- Set up the Code: Create a new file in your code editor and insert the provided code:
import google.generativeai as genai
import PIL.Image
img = PIL.Image.open('image.jpg')
genai.configure(api_key='PASTE YOUR API KEY HERE')
model = genai.GenerativeModel('gemini-pro-vision')
response = model.generate_content(["what is the total calorie count?", img])
print(response.text)
- Add Your API Key: Replace ‘PASTE YOUR API KEY HERE’ with your actual Gemini API key. This code uses the ‘
gemini-pro-vision
‘ model, which combines text and vision capabilities.
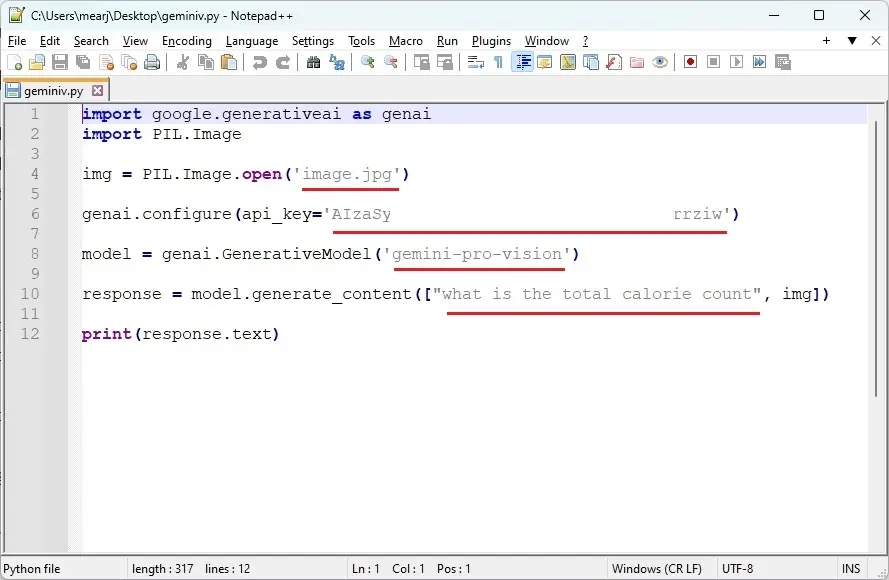
- Save the File: Save the file on your Desktop with a
.py
extension. For example, name itgeminiv.py
.
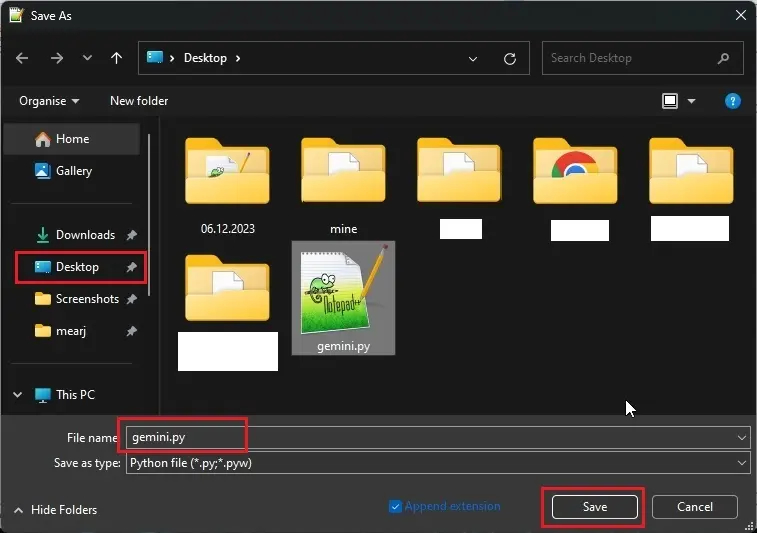
- Specify Image Location: Ensure that an image named
image.jpg
(or the specified image filename) is saved in the same location as thegeminiv.py
file. This code processes a local JPG or PNG file up to 4MB.
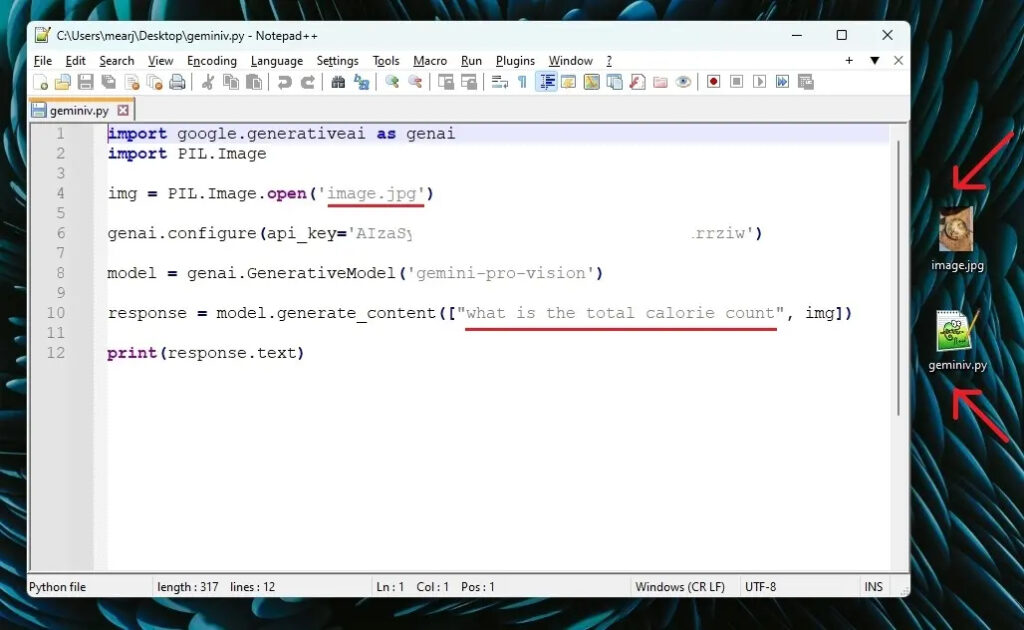
- Ask Questions Related to the Image: Modify the question in the code according to the image content. For example, if using a food-related image, query the Gemini Pro to calculate the total calorie count.
- Execute the Code: Navigate to the Desktop in the Terminal and run the following commands:
cd Desktop
python geminiv.py
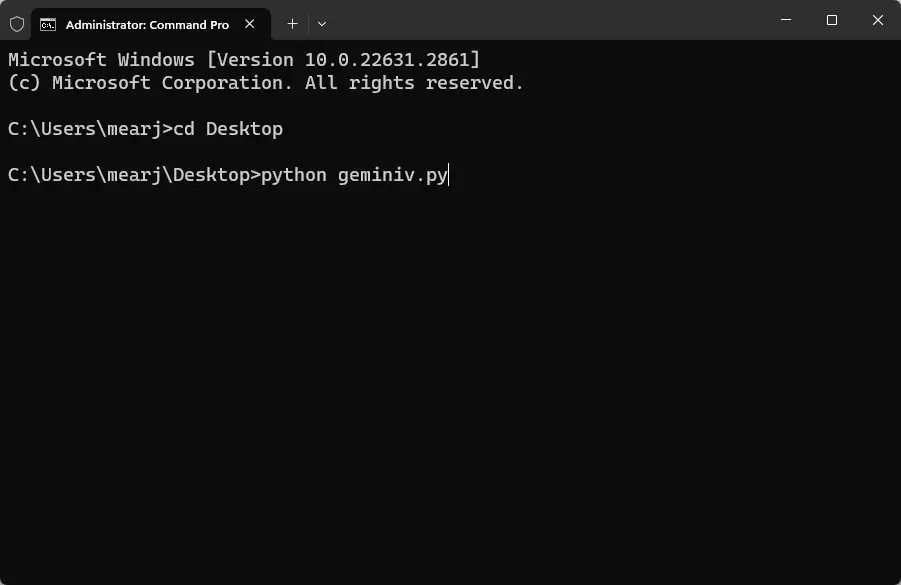
- Observe the Response: The Gemini Pro multimodal model will provide an answer to the question based on the image content. You can further inquire and request explanations from the AI.
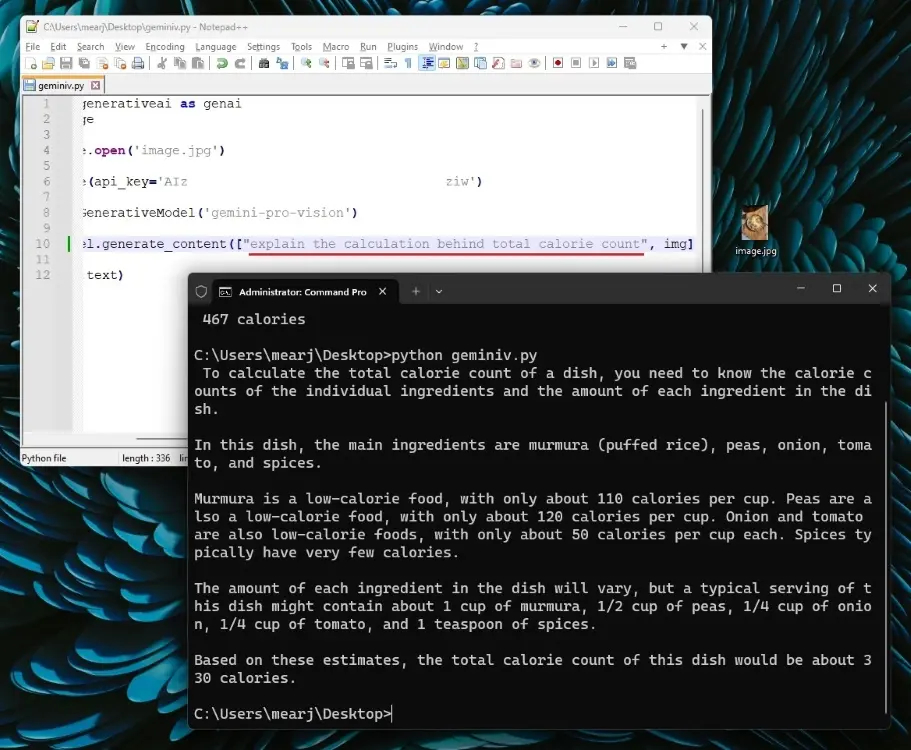
- Try Different Images and Questions: Replace the image with another image file in the same location, adjust the filename in the code, modify the question related to the new image, save the changes, and rerun the
geminiv.py
file to receive new responses based on the new image content and queries.
How to Utilize the Gemini Pro API Key in Chat Format
Unconv’s (GitHub) streamlined code enables direct interaction with the Gemini Pro model through a Terminal window using a Gemini AI API key. This method eradicates the need to modify the question in the code and repeatedly rerun the Python file for updated outputs. The conversation seamlessly continues within the Terminal window.
Moreover, Google’s integration of chat history eliminates the necessity for manual management of response appends or maintaining conversation history in arrays or lists. Through a straightforward function, Google automatically stores the entire conversation history within a chat session. Let me delve into how this process operates.
- Set Up the Code: Copy and paste the given code into your code editor:
import google.generativeai as genai
genai.configure(api_key='PASTE YOUR API KEY HERE')
model = genai.GenerativeModel('gemini-pro')
chat = model.start_chat()
while True:
message = input("You: ")
response = chat.send_message(message)
print("Gemini: " + response.text)
- Insert Your API Key: Replace ‘PASTE YOUR API KEY HERE‘ with your actual Gemini API key.
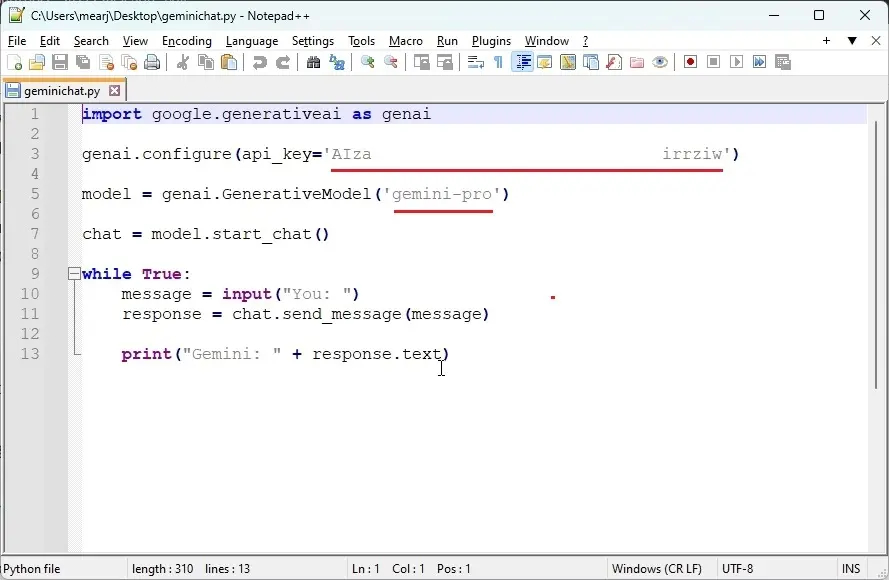
- Save the File: Save the file, adding a
.py
extension, such asgeminichat.py
, to your preferred location (e.g., Desktop).
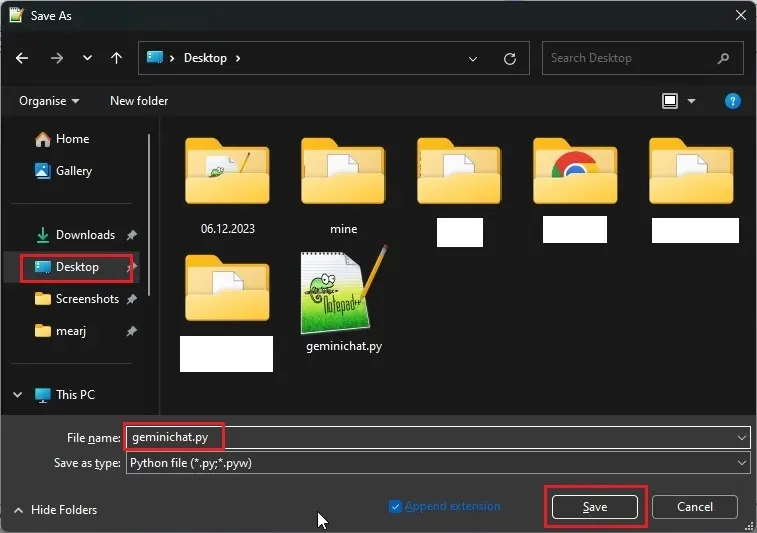
- Access the Terminal: Launch the Terminal and navigate to the location where the
geminichat.py
file is saved (e.g., Desktop).
cd Desktop
- Execute the Python Script: Run the
geminichat.py
file in the Terminal:
python geminichat.py
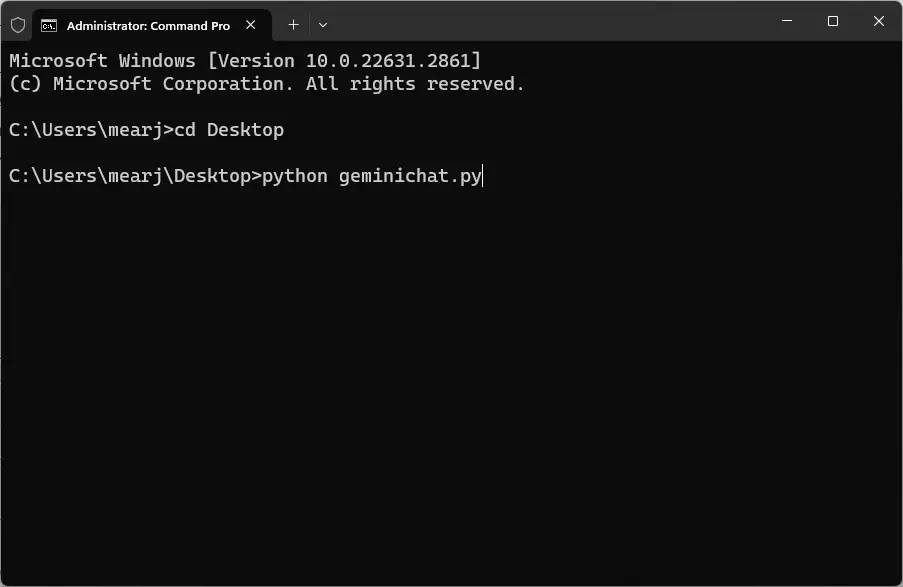
- Engage in Conversation: You can now effortlessly continue the conversation directly within the Terminal. The chat history will also be retained, making it a convenient way to leverage the Google Gemini API key for conversational interactions with the Gemini Pro model.
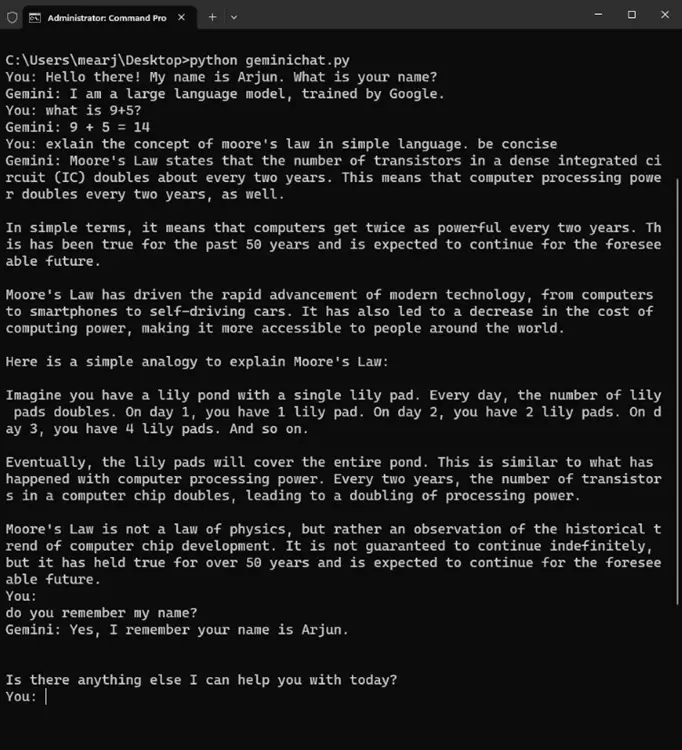
It’s fascinating to explore Google Gemini’s capabilities through its API with these examples. Comparing it to OpenAI’s DALL-E 3 and ChatGPT, it’s interesting how Google has made its vision model accessible for enthusiasts and developers, despite not surpassing the GPT-4V model. The upcoming launch of Gemini Ultra, said to be on par with the GPT-4 model, holds promise for even more advanced capabilities.
There’s a noticeable distinction between the responses from the Gemini Pro API and those from Google Bard, which is also fueled by a refined version of Gemini Pro. Bard’s responses might appear somewhat mundane and sterilized, whereas Gemini Pro’s API responses seem more vibrant and infused with character.
We’ll be closely monitoring developments in this space and will continue to provide updates on Gemini AI-related content. Meanwhile, feel free to explore the Google Gemini API firsthand and witness its capabilities. Stay tuned for more!
0 Comments